When developing multiple python packages and codes, or working with multiple directories, we often come across the __ init __.py
file in Python programming language. In this article, we will learn, what is __ init __.py
file? Also, we will learn about Python packages and also python programming language directory structure.
Table Of Contents
What is a directory?
A directory is a collection of folders/directories, subdirectories, and files, where user often work is known as directories. Below is an image of directory. Here you can see we have a __ init __.py
file.
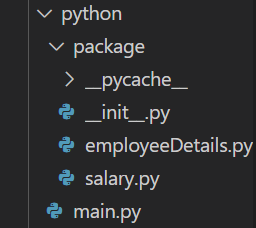
You can see, here we have a directory name python. In this directory we have one package folder in which we have three files:
__ init __.py
- employeeDetails.py
- salary.py
At the root level of directory folder we have main.py
, where we will execute our code. This is the structure of the code we are using in this tutorial.
Module and Package in Python
A module is a Python script, where we have our code for any functionality. Whereas, a Package is collection of different modules where we have different modules for different functionalities.
Types of Packages
Packages are also of two types:
Frequently Asked:
- Replace instances of a character in a String in Python
- How to add items from a list to another list in Python?
- Pandas: Select columns based on conditions in dataframe
- Remove String before a Specific Character in Python
- Regular Packages: This type of packages was used in Python 3.2 and before. This is the type of Package which has a
__ init __.py
file, which is executed as a directory which contains a__ init __.py
file. - Namespace Packages: Here we don’t have
__ init __.py
file, this package is mostly used for large collection of packages. This allows us to divide the sub-package and modules within a single package across multiple packages.
Also __ init __.py
(regular packages) has become optional form python 3.3 but still this the most and recommended and widely used.
Now back to our question,
What is __ init __.py
?
When Python interpreter finds a __ init __.py
file in any directory, then Python interpreter considers this directory as a Python Package. This package can be used in our code after importing it. The __init__.py
file can be blank, but the suggested way is to import all the packages in this __ init __.py
file. After creating this __ init __.py
file, you can import the whole folder(here which is package) and then you can import the other modules of the package(here they are salary.py and employeeDetails.py).
See the example code below and then we will move ahead and understand the __init__.py
and the requirement of __init__.py
in Python Programming language.
Code Examples :
File: employeeDetails.py
# Created a function with employee details. def details(name, age, profile): name = str(name) age = int(age) profile = str(profile) # capitalize() method makes the first character capital. name = name.capitalize() # lstrip() removes any leading whitespace. name = name.lstrip() # lstrip() any whitespaces at last. profile = profile.rstrip() profile = profile.capitalize() print('Name of the Employee: ',name,',Age of the Employee: ', age, ',profile is :', profile )
File: salary.py
# A simple function to generate salary def salary(base): base = int(base) hra = base / 100 * 20 ta = base / 100 *10 da = base / 100 * 7 salary = hra+ta+da+base print('Salary of the Employee is:' , salary)
File: __ init __.py
from package.salary import salary from package.employeeDetails import details
File: main.py
import package name = 'nikhil' base = 20000 age = 25 profile = 'developer' package.details(name, age, profile) package.salary(base)
OUTPUT :
Name of the Employee: Nikhil ,Age of the Employee: 25 ,profile is : Developer Salary of the Employee is: 27400.0
In the above code sample, see we have imported all the packages in the __ init __.py
and then imported the whole package folder as a single package. This is the benefit of the __ init __.py
file, because of this Python Interpreter treats this folder as a Python Package and the files inside it as modules. So you can import the modules inside your main code.
You may also notice a .pyc file after you import something from other module for the first time. It is actually compiled byte code of the imported module.py file which is created by Python interpreter after you first time import any other module. You can consider this as a cache file, next time when you will run the code then the Python Interpreter will first check for the .pyc file, if not found then it will first compile the module into bytecode which you are importing in your module.
Summary
So in this Python article we learned about __ init __.py
file, which turns a directory into an initialization point and the Python Interpreter treats this as a package. All the scripts/code files in the directory will be treated as a module and can be imported and executed hassle-free. Also we learned about modules and packages and its types.
But there are also few things to keep in mind like the directory in which __ init __.py
file should be the root project directory and is set as default to PYTHON PATH variable and Python Interpreter. Like here in the image above you can see we have a directory python in which there is a directory named as python and also a main.py file. Inside the package directory we have __ init __.py
file along with several other python files. Here, python is the root folder and is accessible to the Python Interpreter and PYTHON PATH variable. If not done so, at the time of execution code it will throw an error ModuleNotFoundError
or Import Error
.
Make sure to read the code and directory structure properly, in order to have a better understanding. Thanks.